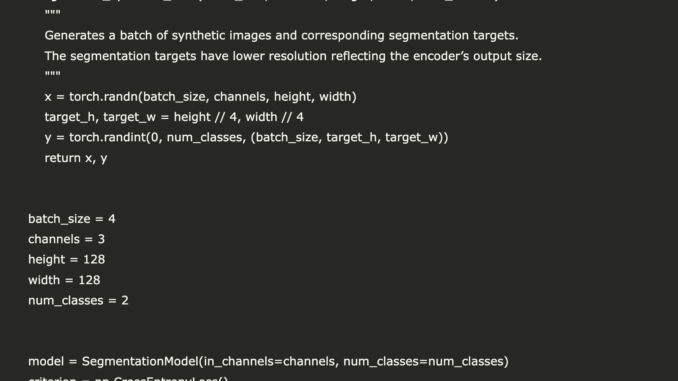
In this tutorial, we explore a novel deep learning approach that combines multi-head latent attention with fine-grained expert segmentation. By harnessing the power of latent attention, the model learns a set of refined expert features that capture high-level context and spatial details, ultimately enabling precise per-pixel segmentation. Throughout this implementation, we will walk you through an end-to-end implementation using PyTorch on Google Colab, demonstrating the key building blocks, from a simple convolutional encoder to the attention mechanisms that aggregate critical features for segmentation. This hands-on guide is designed to help you understand and experiment with advanced segmentation techniques using synthetic data as a starting point.
import torch.nn as nn
import torch.nn.functional as F
import matplotlib.pyplot as plt
import numpy as np
torch.manual_seed(42)
We import essential libraries such as PyTorch for deep learning, numpy for numerical computations, and matplotlib for visualization, setting up a robust environment for building neural networks. Aldo, torch.manual_seed(42) ensures reproducible results by fixing the random seed for all torch-based random number generators.
“””
A basic CNN encoder that extracts feature maps from an input image.
Two convolutional layers with ReLU activations and max-pooling are used
to reduce spatial dimensions.
“””
def __init__(self, in_channels=3, feature_dim=64):
super().__init__()
self.conv1 = nn.Conv2d(in_channels, 32, kernel_size=3, padding=1)
self.conv2 = nn.Conv2d(32, feature_dim, kernel_size=3, padding=1)
self.pool = nn.MaxPool2d(2, 2)
def forward(self, x):
x = F.relu(self.conv1(x))
x = self.pool(x)
x = F.relu(self.conv2(x))
x = self.pool(x)
return x
The SimpleEncoder class implements a basic convolutional neural network that extracts feature maps from an input image. It employs two convolutional layers combined with ReLU activations and max-pooling to progressively reduce the spatial dimensions, thus simplifying the image representation for subsequent processing.
“””
This module learns a set of latent vectors (the experts) and refines them
using multi-head attention on the input features.
Input:
x: A flattened feature tensor of shape [B, N, feature_dim],
where N is the number of spatial tokens.
Output:
latent_output: The refined latent expert representations of shape [B, num_latents, latent_dim].
“””
def __init__(self, feature_dim, latent_dim, num_latents, num_heads):
super().__init__()
self.num_latents = num_latents
self.latent_dim = latent_dim
self.latents = nn.Parameter(torch.randn(num_latents, latent_dim))
self.key_proj = nn.Linear(feature_dim, latent_dim)
self.value_proj = nn.Linear(feature_dim, latent_dim)
self.query_proj = nn.Linear(latent_dim, latent_dim)
self.attention = nn.MultiheadAttention(embed_dim=latent_dim, num_heads=num_heads, batch_first=True)
def forward(self, x):
B, N, _ = x.shape
keys = self.key_proj(x)
values = self.value_proj(x)
queries = self.latents.unsqueeze(0).expand(B, -1, -1)
queries = self.query_proj(queries)
latent_output, _ = self.attention(query=queries, key=keys, value=values)
return latent_output
The LatentAttention module implements a latent attention mechanism where a fixed set of latent expert vectors is refined via multi-head attention using projected input features as keys and values. In the forward pass, these latent vectors (queries) attend to the transformed input, resulting in refined expert representations that capture the underlying feature dependencies.
“””
For fine-grained segmentation, each pixel (or patch) feature first projects into the latent space.
Then, it attends over the latent experts (the output of the LatentAttention module) to obtain a refined representation.
Finally, a segmentation head projects the attended features to per-pixel class logits.
Input:
x: Flattened pixel features from the encoder [B, N, feature_dim]
latent_experts: Latent representations from the attention module [B, num_latents, latent_dim]
Output:
logits: Segmentation logits [B, N, num_classes]
“””
def __init__(self, feature_dim, latent_dim, num_heads, num_classes):
super().__init__()
self.pixel_proj = nn.Linear(feature_dim, latent_dim)
self.attention = nn.MultiheadAttention(embed_dim=latent_dim, num_heads=num_heads, batch_first=True)
self.segmentation_head = nn.Linear(latent_dim, num_classes)
def forward(self, x, latent_experts):
queries = self.pixel_proj(x)
attn_output, _ = self.attention(query=queries, key=latent_experts, value=latent_experts)
logits = self.segmentation_head(attn_output)
return logits
The ExpertSegmentation module refines pixel-level features for segmentation by first projecting them into the latent space and then applying multi-head attention using the latent expert representations. Finally, it maps these refined features through a segmentation head to generate per-pixel class logits.
“””
The final model that ties together the encoder, latent attention module,
and the expert segmentation head into one end-to-end trainable architecture.
“””
def __init__(self, in_channels=3, feature_dim=64, latent_dim=64, num_latents=16, num_heads=4, num_classes=2):
super().__init__()
self.encoder = SimpleEncoder(in_channels, feature_dim)
self.latent_attn = LatentAttention(feature_dim=feature_dim, latent_dim=latent_dim,
num_latents=num_latents, num_heads=num_heads)
self.expert_seg = ExpertSegmentation(feature_dim=feature_dim, latent_dim=latent_dim,
num_heads=num_heads, num_classes=num_classes)
def forward(self, x):
features = self.encoder(x)
B, F, H, W = features.shape
features_flat = features.view(B, F, H * W).permute(0, 2, 1)
latent_experts = self.latent_attn(features_flat)
logits_flat = self.expert_seg(features_flat, latent_experts)
logits = logits_flat.permute(0, 2, 1).view(B, -1, H, W)
return logits
The SegmentationModel class integrates the CNN encoder, the latent attention module, and the expert segmentation head into a unified, end-to-end trainable network. During the forward pass, the model encodes the input image into feature maps, flattens and transforms these features for latent attention processing, and finally uses expert segmentation to produce per-pixel class logits.
x_dummy = torch.randn(2, 3, 128, 128)
output = model(x_dummy)
print(“Output shape:”, output.shape)
We instantiate the segmentation model and pass a dummy batch of two 128×128 RGB images through it. The printed output shape confirms that the model processes the input correctly and produces segmentation maps with the expected dimensions.
“””
Generates a batch of synthetic images and corresponding segmentation targets.
The segmentation targets have lower resolution reflecting the encoder’s output size.
“””
x = torch.randn(batch_size, channels, height, width)
target_h, target_w = height // 4, width // 4
y = torch.randint(0, num_classes, (batch_size, target_h, target_w))
return x, y
batch_size = 4
channels = 3
height = 128
width = 128
num_classes = 2
model = SegmentationModel(in_channels=channels, num_classes=num_classes)
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=1e-3)
num_iterations = 100
model.train()
for iteration in range(num_iterations):
x_batch, y_batch = generate_synthetic_data(batch_size, channels, height, width, num_classes)
optimizer.zero_grad()
logits = model(x_batch) # logits shape: [B, num_classes, H/4, W/4]
loss = criterion(logits, y_batch)
loss.backward()
optimizer.step()
if iteration % 10 == 0:
print(f”Iteration {iteration}: Loss = {loss.item():.4f}”)
We define a synthetic data generator that produces random images and corresponding low-resolution segmentation targets to match the encoder’s output resolution. Then, we set up and train the segmentation model for 100 iterations using cross-entropy loss and the Adam optimizer. Loss values are printed every 10 iterations to monitor training progress.
x_vis, y_vis = generate_synthetic_data(1, channels, height, width, num_classes)
with torch.no_grad():
logits_vis = model(x_vis)
pred = torch.argmax(logits_vis, dim=1) # shape: [1, H/4, W/4]
img_np = x_vis[0].permute(1, 2, 0).numpy()
gt_np = y_vis[0].numpy()
pred_np = pred[0].numpy()
fig, axs = plt.subplots(1, 3, figsize=(12, 4))
axs[0].imshow((img_np – img_np.min()) / (img_np.max()-img_np.min()))
axs[0].set_title(“Input Image”)
axs[1].imshow(gt_np, cmap=’jet’)
axs[1].set_title(“Ground Truth”)
axs[2].imshow(pred_np, cmap=’jet’)
axs[2].set_title(“Predicted Segmentation”)
for ax in axs:
ax.axis(‘off’)
plt.tight_layout()
plt.show()
In evaluation mode, we generate a synthetic sample, compute the model’s segmentation prediction using torch.no_grad(), and then convert the tensors into numpy arrays. Finally, it visualizes the input image, ground truth, and predicted segmentation maps side by side using matplotlib.
In conclusion, we provided an in-depth look at implementing multi-head latent attention alongside fine-grained expert segmentation, showcasing how these components can work together to improve segmentation performance. Starting from constructing a basic CNN encoder, we moved through the integration of latent attention mechanisms and demonstrated their role in refining feature representations for pixel-level classification. We encourage you to build upon this foundation, test the model on real-world datasets, and further explore the potential of attention-based approaches in deep learning for segmentation tasks.
Here is the Colab Notebook. Also, don’t forget to follow us on Twitter and join our Telegram Channel and LinkedIn Group. Don’t Forget to join our 85k+ ML SubReddit.
Asif Razzaq is the CEO of Marktechpost Media Inc.. As a visionary entrepreneur and engineer, Asif is committed to harnessing the potential of Artificial Intelligence for social good. His most recent endeavor is the launch of an Artificial Intelligence Media Platform, Marktechpost, which stands out for its in-depth coverage of machine learning and deep learning news that is both technically sound and easily understandable by a wide audience. The platform boasts of over 2 million monthly views, illustrating its popularity among audiences.
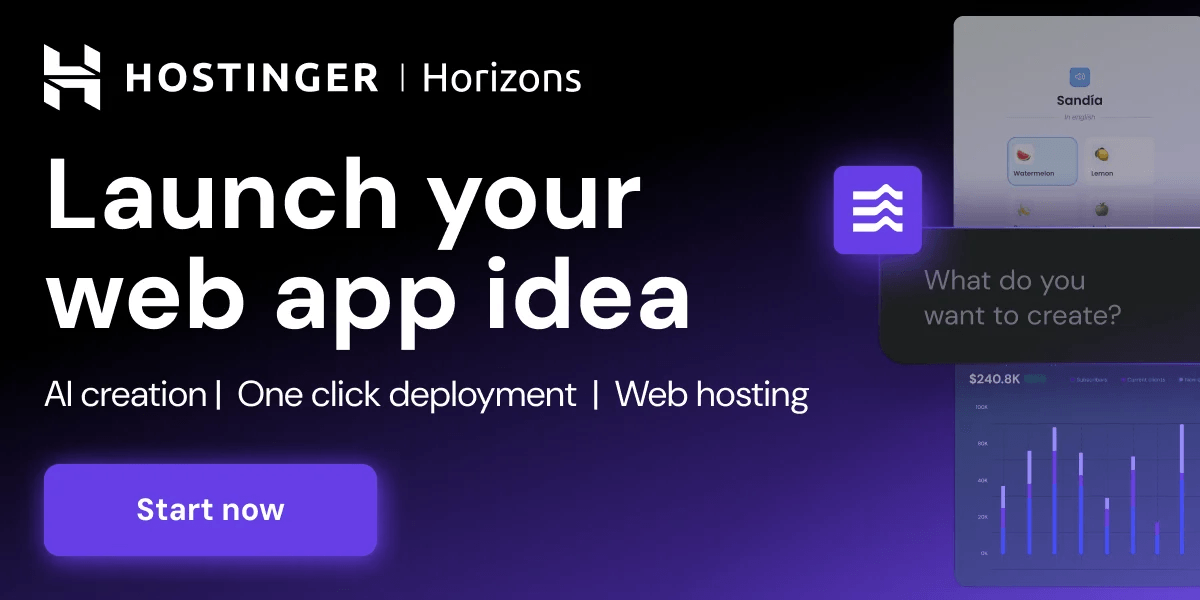
Be the first to comment